TypeScript is a superset of JavaScript that has climbed up in popularity in the past few years. In this post, we will learn how to use optional parameters in TypeScript functions, let’s get started!
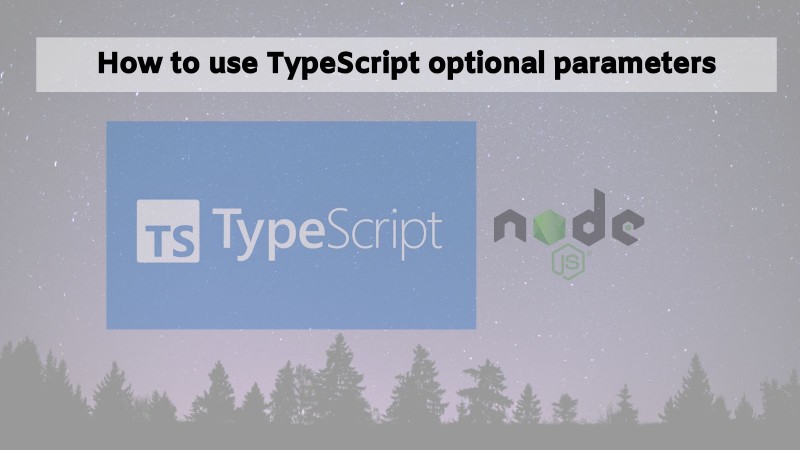
Table of contents #
- Example of printing full name
- Optional parameters in JavaScirpt
- Optional parameters with TypeScript
- Default params in Typescript
- Conclusion
Example of printing full name #
For this tutorial we will use a simple yet useful example of getting the full name, the rules are:
- If the middle name is provided full name has the middle name included
- In case the name has only first and last name the full name will not have the middle name included.
It can be coded as below in both JavaScript and TypeScirpt as we will see in the next section as well as how optional parameters make it easy to get the desired output minimizing errors in TypeScript.
Optional parameters in JavaScirpt #
By default, all the parameters in JavaScript are optional. Let’s look at this with above mentioned example of getting the fullname with first name, middle name and last name below:
function getFullName(firstName, lastName, middleName) {
const fullName = middleName ? `${firstName} ${middleName} ${lastName}` : `${firstName} ${lastName}`;
return fullName;
}
console.log(getFullName());
console.log(getFullName('John'));
console.log(getFullName('John', 'Doe'));
console.log(getFullName('John', 'Doe', 'MiddleName'));
The above code will give the output as follows:
undefined undefined
John undefined
John Doe
John MiddleName Doe
So in ES6 Javascript, there isn’t a way to make a function parameter required, this is how JavaScript works as it is a bit too flexible. That is a reason why we can use TypeScript to add types as well as make the function parameters required, let’s look at the same example with TypeScript. We can use nodemon to compile and execute the script on save. We can also use docker with Node.js to deploy the compiled code easily.
Optional parameters with TypeScript #
For the same example we wil now use TypeScript and write the getFullName
function as below in the optional-params.ts
file, notice the file is .ts
for typescript:
function getFullName(firstName: string, lastName: string, middleName?: string) {
const fullName = middleName ? `${firstName} ${middleName} ${lastName}` : `${firstName} ${lastName}`;
return fullName;
}
//console.log(getFullName('John')); // compile error - An argument for 'lastName' was not provided.
console.log(getFullName('John', 'Doe'));
console.log(getFullName('John', 'Doe', 'MiddleName'));
The
?
after the middle name indicates the TS compiler it is an optional parameter.
We will also need to create a tsconfig.json
file with the following contents:
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"sourceMap": true
}
}
We will use tsc
and node
to run the above TypeScript file after compiling it to JavaScript with the following command:
tsc optional-params.ts && node optional-params.js
Given we have Node installed, we can get the TypeScript compiler with npm install -g typescript
. The above command will first compile the TypeScript file called optional-params.ts
to Javascript and the second command will execute the Javascript as a Node.js script. It will give us the following output:
John Doe
John MiddleName Doe
Let us quickly go through what the above script does:
- The
getFullName
function takes 3 parameters,firstName
,lastName
andmiddleName
where onlymiddleName
is optional. - If there is
middleName
is passed the function will print the full name with the middle name else it will only be first name and last name. - Line 6 if uncommented will stop the compilation process with the error
An argument for 'lastName' was not provided.
as the last name is a required parameter by default. - Line 7 and 8 will work where the first valid call will log
John Doe
without a middle name and the second call will logJohn MiddleName Doe
with the optional parameter of the middle name passed.
You can try the above code in the TypeScript playground and fiddle around with it or use this one.
Default params in Typescript #
The above typescript code can be simplified with use of default params, for instance if the middleName
can be set to ''- empty string if not provided the above code can be written as:
function getFullName(firstName: string, lastName: string, middleName: string = '') {
const fullName = `${firstName} ${middleName} ${lastName}`;
return fullName;
}
//console.log(getFullName('John')); // compile error - An argument for 'lastName' was not provided.
console.log(getFullName('John', 'Doe'));
console.log(getFullName('John', 'Doe', 'MiddleName'));
The above function looks pretty similar the main difference is, that the middleName
is set as a default value of an empty string if not provided. This has a similar output as seen below:
John Doe
John MiddleName Doe
There is a couple of extra spaces when only the first name and last name are printed but that is a tradeoff we can take for the simplified code. All the code is available as part of this pull request for your reference.
Conclusion #
In this useful guide, we saw how to use optional parameters in the TypeScript function with a simple yet practical example.
I hope it has helped you unravel how optional and default parameters work in TypeScript.
Keep learning!